According to me Selenium is one of the best open source, free tool for testing web applications. It's quite powerful and gives you the facility to write down your test case in your favorite language ( from the plenty of languages it supports ). But it sucks when it comes to handle the dialogs. Dialog boxes are like ghosts to selenium, It can't even see them.
That's because dialogs are native to the operating system you are running and selenium are designed to identify only web component.
After searching too much on the Internet and other forums, I finally got the solution to all my problems. The solution is too simple. As you can't handle the dialog box through selenium, use the other software to do this. And AutoIT is best at this.
Download Execuable (exe) to Handle Save Dialog of Firefox
Download Execuable (exe) to Handle Save Dialog of Internet Explorer
Download Execuable (exe) to Handle Authentication Dialog
AutoIt
Autoit is a free scripting language designed to automate the windows component. It's just like an extensive VB Script and it allows you to convert the script in executable (exe). So what you can do is, write down the script to handle dialog box using AutoIt, convert it into executable and then call the executable when required. So let's see guys how to do it. But before we begin you need to download and install the Autoit. Download it from the following link
Download AutoIt
Handing Modal Dialogs
Modal Dialog box mean once it has appeared all the focus of that application will be on that dialog box only. You will not able to access any of the other component of parent window until you close the dialog box.
Some of the modal dialog boxes that we face frequently during web application testing are
Save Dialog Box
Save dialog box is bit different in IE and in Firefox. So I have write down two separate script for that.
AutoIt script to handle Firefox Save Dialog box
In Firefox when you click on the button/link to download a file. First it will ask you for you permission once you select yes, If you have selected to ask you for the path then only a dialog box will display.
If you will click on download link following dialogbox will appear in Firfox.
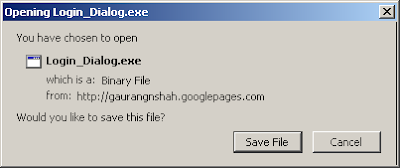
If you click "Save File" on above dialogbox this dialog box will apppears, Yeah if you have choose to ask where to save file from Option.
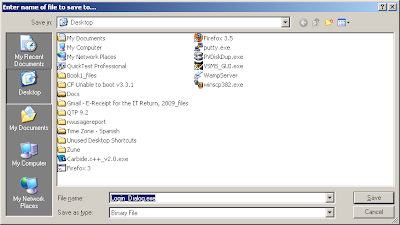
The executable and the script below will handle both of these dialogboxes. You just need to call it in proper way. Your need to pass arguments while calling the script or executable as following.
Save_Dialog_FF Operation FilePath
Operaion: Operation you want to perform when dialog box appears. Can be any of the following
cancel: Press Cancel on the dialog box
Save: Press the Save as Dialog box
FilePath: It's an option argument. It's a full path where you want to save the file.
i.e. C:\myfile.txt
AutoIt script to handle Internet Explorer Save Dialog box
Unlike Firefox dialog box internet explorers dialog box is bit different. First dialog box prompts with three choices Run, Save and cancel. The second dialog box is same as Firefox. If you click on the download link in the internet explorer following dialog box will display.
If you will click on "Save" button on the above dialog box Following dialog box will appear which will ask for the path to save the file.
The executable and the script below will handle both of these dialog boxes. You just need to call it in proper way. Your need to pass arguments while calling the script or executable as following. Save_Dialog_IE Operation FilePath Operaion: Operation you want to perform when dialog box appears. Can be any of the following Run: Clicks on the Run button. cancel: Press Cancel on the dialog box Save: Press the Save as Dialog box FilePath: It's an option argument. It's a full path where you want to save the file. i.e. C:\myfile.txt
Now you just need to copy and paste above scripts in Autoit IDE, convert those into executables, Save those to the project folder. And then just make a call to the executables with proper arguments, just before you execute the step which invokes the dialog box as shows here.
User Name and Password Dialog Box.
following is the AutoIt script to handle to User name and password dialog box.
That's because dialogs are native to the operating system you are running and selenium are designed to identify only web component.
After searching too much on the Internet and other forums, I finally got the solution to all my problems. The solution is too simple. As you can't handle the dialog box through selenium, use the other software to do this. And AutoIT is best at this.
Download Execuable (exe) to Handle Save Dialog of Firefox
Download Execuable (exe) to Handle Save Dialog of Internet Explorer
Download Execuable (exe) to Handle Authentication Dialog
AutoIt
Autoit is a free scripting language designed to automate the windows component. It's just like an extensive VB Script and it allows you to convert the script in executable (exe). So what you can do is, write down the script to handle dialog box using AutoIt, convert it into executable and then call the executable when required. So let's see guys how to do it. But before we begin you need to download and install the Autoit. Download it from the following link
Download AutoIt
Handing Modal Dialogs
Modal Dialog box mean once it has appeared all the focus of that application will be on that dialog box only. You will not able to access any of the other component of parent window until you close the dialog box.
Some of the modal dialog boxes that we face frequently during web application testing are
Save Dialog Box
Save dialog box is bit different in IE and in Firefox. So I have write down two separate script for that.
AutoIt script to handle Firefox Save Dialog box
In Firefox when you click on the button/link to download a file. First it will ask you for you permission once you select yes, If you have selected to ask you for the path then only a dialog box will display.
If you will click on download link following dialogbox will appear in Firfox.
If you click "Save File" on above dialogbox this dialog box will apppears, Yeah if you have choose to ask where to save file from Option.
The executable and the script below will handle both of these dialogboxes. You just need to call it in proper way. Your need to pass arguments while calling the script or executable as following.
Save_Dialog_FF Operation FilePath
Operaion: Operation you want to perform when dialog box appears. Can be any of the following
cancel: Press Cancel on the dialog box
Save: Press the Save as Dialog box
FilePath: It's an option argument. It's a full path where you want to save the file.
i.e. C:\myfile.txt
;--------------------------------------------------------- ;~ Save_Dialog_FF.au3 ;~ Purpose: To handle the Dowload/save Dialogbox in Firefox ;~ Usage: Save_Dialog_FF.exe "Dialog Title" "Opetaion" "Path" ;~ Create By: Gaurang Shah ;~ Email: gaurangnshah@gmail.com ;---------------------------------------------------------- ; set the select mode to select using substring AutoItSetOption("WinTitleMatchMode","2") if $CmdLine[0] < 2 then ; Arguments are not enough msgbox(0,"Error","Supply all the Arguments, Dialog title,Save/Cancel and Path to save(optional)") Exit EndIf ; wait until dialog box appears WinWait($CmdLine[1]) ; match the window with substring $title = WinGetTitle($CmdLine[1]) ; retrives whole window title WinActive($title); ; if user choose to save file If (StringCompare($CmdLine[2],"Save",0) = 0) Then WinActivate($title) WinWaitActive($title) Sleep(1) ; If firefox is set the save the file on some specific location without asking user. ;Save the File send("{TAB}") Send("{ENTER}") if ( StringCompare(WinGetTitle("[active]"),$title,0) = 0 ) Then WinActivate($title) send("{TAB}") Send("{ENTER}") EndIf ;if firefox is set to prompt user for save path. if WinExists("Enter name") Then $title = WinGetTitle("Enter name") if($CmdLine[0] = 2) Then ; If user hasn't provided path to save ;save to default path. WinActivate($title) ControlClick($title,"","Button2") Else ; If user has provided path ;Set path and save file WinActivate($title) WinWaitActive($title) ControlSetText($title,"","Edit1",$CmdLine[3]) ControlClick($title,"","Button2") EndIf Else ;Firefox is configured to save file at specific location Exit EndIf EndIf ; do not save the file If (StringCompare($CmdLine[2],"Cancel",0) = 0) Then WinWaitActive($title) Send("{ESCAPE}") EndIfDownload above script
AutoIt script to handle Internet Explorer Save Dialog box
Unlike Firefox dialog box internet explorers dialog box is bit different. First dialog box prompts with three choices Run, Save and cancel. The second dialog box is same as Firefox. If you click on the download link in the internet explorer following dialog box will display.
;-------------------------------------------------------------------- ;~ Save_Dialog_IE.au3 ;~ Purpose: To handle the Dowload/save Dialogbox in Internet Explorer ;~ Usage: Save_Dialog_IE.exe "Dialog Title" "Opetaion" "Path" ;~ Create By: Gaurang Shah ;~ Email: gaurangnshah@gmail.com ;-------------------------------------------------------------------- AutoItSetOption("WinTitleMatchMode","2") ; set the select mode to select using substring if $CmdLine[0] < 2 then ; Arguments are not enough msgbox(0,"Error","Supply all the arguments, Dialog title,Run/Save/Cancel and Path to save(optional)") Exit EndIf ; wait Until dialog box appears WinWait($CmdLine[1]) ; match the window with substring $title = WinGetTitle($CmdLine[1]) ; retrives whole window title WinActivate($title) If (StringCompare($CmdLine[2],"Run",0) = 0) Then WinActivate($title) ControlClick($title,"","Button1") EndIf If (StringCompare($CmdLine[2],"Save",0) = 0) Then WinWaitActive($title) ControlClick($title,"","Button2") ; Wait for the new dialogbox to open Sleep(2) WinWait("Save") $title = WinGetTitle("Save") ;$title = WinGetTitle("[active]") if($CmdLine[0] = 2) Then ;click on the save button WinWaitActive($title) ControlClick($title,"","Button2") Else ;Set path and save file WinWaitActive($title) ControlSetText($title,"","Edit1",$CmdLine[3]) ControlClick($title,"","Button2") EndIf EndIf If (StringCompare($CmdLine[2],"Cancel",0) = 0) Then WinWaitActive($title) ControlClick($title,"","Button3") EndIfDownload above script
Now you just need to copy and paste above scripts in Autoit IDE, convert those into executables, Save those to the project folder. And then just make a call to the executables with proper arguments, just before you execute the step which invokes the dialog box as shows here.
/** * @author Gaurang Shah * Purpose: Shows how to invoke Executable to handle dialog box * Email: gaurangnshah@gmail.com */ import org.testng.annotations.*; import com.thoughtworks.selenium.DefaultSelenium; import com.thoughtworks.selenium.Selenium; public class Handle_Dialogs { private Selenium selenium; @BeforeClass public void startSelenium() { selenium = new DefaultSelenium("localhost", 4444, "*chrome", "http://www.autoitscript.com/"); selenium.start(); } @AfterClass(alwaysRun=true) public void stopSelenium() { this.selenium.stop(); } @Test public void handleSaveDialog() throws Exception { String[] dialog; selenium.open("/site/autoit/downloads/"); selenium.waitForPageToLoad("3000"); selenium.click("//img[@alt='Download AutoIt']"); Thread.sleep(2000); String browser = selenium.getEval("navigator.userAgent"); if(browser.contains("IE")){ System.out.print("Browser= IE "+browser); dialog = new String[]{ "Save_Dialog_IE.exe","Download","Save" }; Runtime.getRuntime().exec(dialog); } if(browser.contains("Firefox")){ System.out.print("Browser= Firefox "+browser); dialog = new String[] { "Save_Dialog_FF.exe","Opening","save" }; Runtime.getRuntime().exec(dialog); } } }Download above File
User Name and Password Dialog Box.
following is the AutoIt script to handle to User name and password dialog box.
;~ ------------------------------------------------------------ ;~ Save_Dialog_IE.au3 ;~ To handle the Authentication Dialogbox ;~ Create By: Gaurang Shah ;~ Usage: Login_dialog.au3 "Dialog Title" "User Name" "Password" ;~ ------------------------------------------------------------ AutoItSetOption("WinTitleMatchMode","2") if $CmdLine[0] < 3 then msgbox(0,"Error","Supply all the Arguments, Dialog title User Name, Password") Exit EndIf WinWait($CmdLine[1]) ; match the window with substring $title = WinGetTitle($CmdLine[1]) ; retrives whole window title ControlSend($title,"","Edit2",$CmdLine[2]);Sets User Name ControlSend($title,"","Edit3",$CmdLine[3]);Sets Password ControlClick($title,"","OK");Download above script
64 comments:
Hi Gaunrang,
Really thanks for nice article.
I have two radio buttons on modal dialog. By default Open radio button is selected. i want to select Save radio button and click Ok button.
Can you put some light on above approach.
Thanks
I just had a similar issue where a script that had a pop-up containing a checkbox that I wasn't expecting. When I incremented over the Buttons, one of them was the checkbox. I believe that radio buttons and checkboxes are considered "buttons" when trying to access them. Try ControlClick($title,"","Button#") as in the examples above until you find the button that clicks the correct radio.
i have an issue , i'm new to selenium and i'm trying selenum automation using php and selenium rc . I want selenium to click on a button. how can i do this using php. thanks
Hi thomas,
What kind of button is this ? Is it web button or win button ?
If its a web button, Record the script through selenium and then export it in php, you might require little modification to make it run. Check out my other posts on selenium for recording.
If it's a win button. Write down the script in autoit and then convert it into exe. Call the exe or script before you execute the step where win button appears.
To execute exe through php use something like this:
$result = shell_exec("program.exe");
i want to click on a download dialog in firefox using selenium rc and php. i'm own a mac so i cant run autoit
hi i'm using firefox, selenium rc and php. I want to automate selenium so it clicks on a firefox download dialog box. Is this possible?
Hi Thomas,
Check out the following post.
http://qtp-help.blogspot.com/2009/08/selenium-handling-dialog-box-in-mac.html
Hi,
on the save dailog box... defaultly "open" checkbox is checked . so, when i ran the autoit script download file is saving in some temp location eventhough i have specified the full path . could you please help to save the file in some particular location.
Hi Gaurang,
This is regarding the type=file problem in IE. It's working fine in firefox but I am not able to give the file type parameter in IE RC.
I'm having an intermitent problem where the save dialog box appears to not be activating. save_file has recognised that the dialog box is there but will not activate it or save the file. Has anyone else come accross this?
Hi Gaurang,
Nice article .. I was struggling with authentication dialog box as I did not know the control ids for username and password. Finally managed to do it with little modification to your script by using Send("{TAB}"} key :)
I have an issue I have recorded my code thru selenium and that code is working with firefox but i want that code ahould work with IE as well. Now the only problem is that when im runnung the code after automating it there is one option in which a pop up window gets open and we have to click on all the check boxes by clicking on check all. It is not clicking on the check box but filling up rest of the form.
Hi,
While automating my application i have one problem with alert box.I keep the mandatory field blank in my application and i will click on the save button at that time alert message will pop up for the mandatory fields.How to handle this case in selenium.
Hi Nagraj,
I guess it's inputbox you are talking about. What you can do is you can write down small script autoit which identifies and focus the input box and fill the data.
Alternately you can also you the Robot API of JAVA.
Hi Gaunrang,
Thanks for the great article. I'm trying to implement what you wrote about - but in a Selenium Grid environment. I'm not sure if its possible - do you have any advice on how to do this - or if its possible?
Thanks
Hey Gaunrang,
Your firefox script is extremely helpful. However, it seems to fail when I try saving in a path with blank spaces eg: "C:\Documents and Settings\..." , it works fine otherwise. Maybe I'm not putting in the parameters correctly. I would appreciate your help. Thanks!
Kabeer
Hi Gaunrang,
I have a some code lines below:
-line 1: selenium.Open(InitUrl);
-line 2: System.Diagnostics.ProcessStartInfo procStartInfo = new System.Diagnostics.ProcessStartInfo("cmd", "/C" + "Login_Dialog.exe" + "\"Connect to crmtestmachine\"" + "username password");
...
-line n: System.Diagnostics.Process proc = new System.Diagnostics.Process();
...
-line n+1 : proc.StartInfo = procStartInfo;
-line n+2 : proc.Start();
I try to execute command line to run Login_Dialog.exe
BUT selenium executed line 1 and timed out after 30000ms(default of selenium)
I cannot send command line. Please help me.
Thanks!
Son Nguyen
Hi Gaurang,
Thanks for sharing this workaround. For some reason I got no success using ControlSend and ControlClick commands, but work around it by using key board controls like Send("{ASC 117}"), Send("{TAB}") and Send("{ENTER}").
Compile following autoit code (say as Login.exe)
;**********************
If WinExists("Authentication Required") Then
WinSetOnTop("Authentication Required", "", 1)
WinActivate("Authentication Required", "")
; to set the username
Send("{ASC 117}") ; u
Send("{ASC 115}") ; s
Send("{ASC 101}") ; e
Send("{ASC 114}") ; r
; to set the password
Send("{TAB}") ; now at password
Send("{ASC 112}") ; p
Send("{ASC 97}") ; a
Send("{ASC 115}") ; s
Send("{ASC 115}") ; s
Send("{TAB}") ; control on OK button
Send("{ENTER}") ; click OK
EndIf
;**********************
And in each Selenium test, start the Login.exe process. See sample below.
[SetUp]
public void SetupTest()
{
selenium = new DefaultSelenium("localhost", 4444, "*firefox", "http://replaceURL/");
selenium.Start();
Process.Start("C:\\Login.exe");
verificationErrors = new StringBuilder();
}
Hope this would help someone.
Thanks,
VJ
HiGaurang,
I am using selenium to automate a webpage.It contains the flash ui.When I am trying to locate the reference id by using the Firebug plugin from firefox it's not locating the flash and when I am trying to record from selenium IDE also the same problem.Is there anyway to handle the flsh componants from Selenium RC?
Thanks in Advance
Kumar
Hi Suman,
To automate the flash content with selenium you would require Flash java client. check out the following link. it might help you.
http://code.google.com/p/flash-selenium/
Thanks Gaurang
Hi Gaurang
i want to call save file dialog script in selenium,how can i do this? and where should i call the script, i am using c# as a language.
is there any way to handle save file dialog without using AutoIt script>
thanks
Hi Gaurang Shah,
This is Ravi from HYD can u plz explain me these examples with C#.
And how to call a AutoIT.exe from C# file.
I think ur developing and using ur framework with java only.
am i right?
Hi Gaurang,
I worked with selenium 1.0 -RC with C#.
My application gets alert messages on final button click. When i run the application -selenium does not display the popup but it does identify the popup and throws its error.
Selenium.SeleniumException: ERROR: There was an unexpected Confirmation!
The line where the popup comes normally is:
selenium.Click("ctl00_C1_btnCalculateCost");
selenium.WaitForPageToLoad("60000"); selenium.ChooseOkOnNextConfirmation();
selenium.Click("ctl00_C1_btnSellPrice"); //alert msg.
if (selenium.IsAlertPresent())
{
selenium.GetAlert();
selenium.Click("//input[@value='OK']");
}
But still the error occurs.
What can be the work around.
Hey happy,
From your code it seems like confirm box is being loaded at pageload event(when you new page opens) if this is the case selenium does not handle the alertbox appears at page load event, I don't know if they have fixed it or not... but this was it few months back !!!
Hi Gaurang,
Really a very nice article.
By default the dialog box opens with radio buttons. How can I save the file using autoit script with these radio buttons. Can you please explain me?
Thanks in advance.
Hi Gaurang,
Really a very nice article.
By default dialog box opens with radio buttons.
Can you please explain me to save the file with these radio buttons using autoit script?
Thanks in advance
Hi Gaurang,
I have a query in Selenium Rc .
input type="text" id="PullQty7390" name="PullQty7390" nvFTrade="True" nvType="PullQty" nvStock="5082" nvRemaining="1" nvMaxValue="1" nvDefaultValue="0" nvOrdered="1" onblur="JavaScript:checkPullBounds(this,1);"
d="PullQty7390 incremented each every time when i run the script from the beginning.How to solve the locator issue ?
use the regular expression
selenium.click("//input[@id='regex:PullQty.*']");
Hi Gaurang
how can i download a file from firefox, selenium is not selecting the ok button on the pop-up
Hello,
I am using C# with selenium RC, Can you please help me for following lines in C# ?
System.out.print ("Browser= IE "+browser);
Runtime.GetRuntime().exec(dialog);
As 'out' and 'runtime' are not available in C#...
Please help.....
hey kartiki,
sysout.out.print is just for debugging purpose it just print the output to screen.
RRuntime.GetRuntime().exec(dialog); is for opening the new process, in our case dialog. There is function is C# for the same. "Process.Start"
Nice article. But i'm facing below issues in firefox 3.6 with windows 7
Save_Dialog_FF.au3 provided here doesn't click the save button after pop up displays to save file.
Also auto It window not displays the window details of Firefox but it does in IE.
So please help me to resolve the issues.
hey dkarunan,
The script here are for few standard dialogs only and tested with firfox 3.6 and IE 8 in windows XP. If it's not working u can make the little changes accordingly.
HI Gaurang Shah
are there any example for file upload box by webdriver ?
thanks
Hi Gaurang,
Is there any way to handle alerts when the duplicate data is fetched from excel sheet. I'm using testNG for running my testcases.
Thanks in advance,
Arun
Hi,
I have a simpler version of your File download dialog box, where I just want to save it with default values in Firefox.
I converted the AutoIT script to an exe and am trying to call it from Java as you are in the example, but it seems to have no effect as the dialog box lingers on. Its as though no code was executed, there is nothing in the Java IDE console or on the command prompt when i execute it that way.
I did add the exe path to the system path. What am I missing?
Thanks for the script, it is very useful.
I have updated it so it works in WinXP/7 with or without RDP. Also removed focus part of script, you should be able to run file download tests locally and perform other tasks without breaking the tests.
I was not able to post full code in the comment, here is the link:
https://docs.google.com/document/d/16wQBe_Q4HvLo3L9RMcSrzCvak-ebidq-eo2VYiURfXQ/edit?hl=en_US
Hi Gaurang,
I am using selenium rc with java.When downloading a file, a new window dialog with two radio buttons(one is open with and other is save file) is appearing.
Can u plz tell me how to handle that dialog box?
Thanks is advance
siri
This is nice article. Would be nice to have discussion topic of how to use (and its feasibility) of AutoIt used in a Selenium Grid environment.
Also, article offers nice language platform independent approach, but for languages that support it, calling AutoIt via native DLL call or COM/ActiveX offers tighter integration rather than externally executing a compiled AutoIt script binary.
Hi Gaurang,
I am very new to selenium RC. I am using selenium Rc with C#. I am facing problem in alert message pop up. In my application when username and password value is incorrect, pop up appears with message "Invalid user name or password" and with "Ok" button. But in my script if i am not using selenium.click command to click on "ok" button of aleet message, still script will run and other values from csv file.
I am fectching data from csv file.
Please help me to solve my query. Thanks in advance.
Hi Gaurang
I am kotresh , present I working on PDF download from selenium webdriver. when I click(from automation script) on PDF icon in my application it opening dialog window with “OK” , “Cancel” and “Browse” button , but when I run autoit script(file name:-Save_Dialog_FF.exe) it clicking on browse button.
public void pdfDownload() throws IOException, InterruptedException {
String[] s = new String[]{"C:/AutoIt/Save_Dialog_FF.exe","Opening ECOB-561.pdf", "Save", "C:\\DownloadLatest\\"};
Runtime.getRuntime().exec(s);
Thread.sleep(4000);
Regards
Kotresh
Hi Gaurang,
Thanks for sharing your experience. I am working on Selenium with Python. As you described that pop up Windows dialog boxes are invisible to Selenium, my project is a web app written in ASP.NET whose authentication is configured in IIS server as Windows authentication. When the app starts, it pops up a login screen to user authentication. I tried to follow your advice to use autoit but it has been without luck. The following is my code fragment for the login part:
url = u"http://www.example.com/"
selrc = MySel("localhost", 5555, "*firefox", url)
selrc.start()
# process login with "autoit"
subprocess.call(["C://Python25//LoginER.exe Authentication admin 1234"])
try:
selrc.open("/Content/Report/ReportData.aspx")
except Exception, e:
print e
I couldn't get autoit to enter the username and password successfully. Could you please kindly shed some light on this?
Regards and thanks
Marvin
Hi Gaurang,
I am from your home town (Ahmedabad), we are using selenium rc with python. I want to check selected folder or file name
is it possible and if yes then how?
Chaitanya
Hi Gaurang,
Hope you are doing well.
I am using Selenium RC with Java. In my application one dialog box with list box and buttons are available. These fields are web elements. Please help me in handling these fields.
Thanks,
Nagesh
Hi Gaurang,
This is Brahmesh from Hyderabad. I have an issue with Webpage dlgs in my appliction. Basically, this page consists of the controls like "Class Code" edit box and "Search" button. After I search for the Class Code with these controls, I have to select the record from the search results with the radio button attached with this and click on the "Select" to get back to the Parent browser window. How do we handle this scenario using AUTOIT? I know AutoIT is fine with Windows Dlgs. But is ther any workaround to handle Webpage dlgs? If so, could you please share the code with me?
Thanks for you help Gaurang!!!!
Regards,
Brahmesh
This is Brahmesh again ....
I have solved this ways .... But do you have other way of solving the same?
If WinExists("Insurance Decisions -- Webpage Dialog") Then
;WinSetOnTop("Insurance Decisions -- Webpage Dialog", "", 1)
WinActivate("Insurance Decisions -- Webpage Dialog", "")
; to set the calss code
Send("6319")
Send("{TAB}")
Send("{ENTER}") ; ; control on Search buttton
Sleep(3000)
Send("{TAB}")
Send("{SPACE}")
Sleep(3000)
Send("{TAB 3}")
Send("{ENTER}")
EndIf
Regards,
Brahmesh
Hi,
Can someone how to automate the scripts using selenium IDE.
In particulars,
I require the perl script to automate it in google chrome.I have tried firefox,it works fine.But in google chrome the browser gets launched and further execution stops by throwing errors that it couldn't find the required xpath elements.
Xpath elements were given correctly and works fines with the firefox.Can anyone help me with the google-chrome
Regards,
Anurag
Hi,
Iam testing an application where in I have to
1. Upload a file
2. Select Accept button in a java dialog box.
The main problem of mine is Iam very much new to Selenium and do not know a,b,c of programming,and been searching for the solution for the last one week.Would really appriciate if any one let me know how to come over this problem,I have just downloaded Autoit but would also like to know how to make call from Selenium.
In hope of a solution.
Thanks in Advance.
Ali
Hi Gaurang,
I have downloaded the Login_dialog.exe and saved it under the project folder and did called it as mentioned above but still it didn't work for me. Could you kindly send me the code how to do it?
Here is the code I used in my selenium script
String args[];
driver.navigate().to(CONFIG.getProperty(object));// opening the URL
args = new String[] { "Login_dialog.exe","username","password" };
Runtime.getRuntime().exec(args);
Hi Gaurang,
i am using the autoit script to save a file. it's working on localhost but when executing it on remote server, Runtime.getRuntime().exec(dialog); gets executed but no action is being done over the window. autoIT is installed on remote server as well as local host.
any suggestion?
Hey Gaurang,
I wanna test my application in terms of user scalability means I have o n number of users to perform the same action on the same tym, the user load supported, the number of transactions, the data volume etc.. Can you suggest me which one of the automation tool can help me.Its web based application and have so many users.
Thanks
Neeraj
Hi Gaurang,
I wanted to use autoIt script in seleniumIDE.is it possible?
I am using Selenuim webdriver, any examples for anyone who has implemented?
Very nice article and helpful.
What I am facing is, I can not get back to selenium browser(IE of FF) to proceed after downloading a file is done.
How to achieve that, please help...
Hi All,
I am implementing this using Selenium Webdriver as well with c#, .net and AutoIt.
When I use System.Diagnostics.ProcessStartInfo(with the arguments mentioned) it states that System.Diagnostics.ProcessStartInfo is a 'type', which is not valid in the given context.
Is ther anyone who has implemented this on c#, AutoIt and webdriverbackedSelenium? Please help. I am really struggling with this.
Any help is really appreciated
Hi, is it possible to do the same with python?
Hi. How I can do it with the new version of firefox. than contain radio buttons.
thanks in advance and thanks for the info.
Hi Gaurang,
Thank you for your sharing. It very useful for me.
I use it successful.
But in case due to any errors, no dialog opened. At that time, the auto script is run and it runs till I exit it.
How can we verify the dialog is opened or not?
Thank you in advance
Hanh
Hi Gaurang,
What is the way to retrieve a file from a folder in java. Lets say, i have a browse button which pops up a window to select a particular zip file from a directory.
Do i have to use any external source for it is it possible through java code only..?
Hi friends,
I have two radio buttons on window popup. By default Open radio button is selected. i want to select Save radio button and click Ok button.
Can you please put some light on above approach.
Thanks
Great Post Man!!!
Hi,
Thanks for the scripts , I downloaded and created executable and included in my project.
worked well !!
Hi,
I am using the selendroid, i am facing the problem on dialog box handling. how to handle dialog box & click ok/cancle button using selendroid script. i am using java as a supportrd language.
Thanks
Nitu
Post a Comment