According to me Selenium is one of the best open source, free tool for testing web applications. It's quite powerful and gives you the facility to write down your test case in your favorite language ( from the plenty of languages it supports ). But it sucks when it comes to handle the dialogs. Dialog boxes are like ghosts to selenium, It can't even see them.
That's because dialogs are native to the operating system you are running and selenium are designed to identify only web component.
After searching too much on the Internet and other forums, I finally got the solution to all my problems. The solution is too simple. As you can't handle the dialog box through selenium, use the other software to do this. And AutoIT is best at this.
Download Execuable (exe) to Handle Save Dialog of Firefox
Download Execuable (exe) to Handle Save Dialog of Internet Explorer
Download Execuable (exe) to Handle Authentication Dialog
AutoIt
Autoit is a free scripting language designed to automate the windows component. It's just like an extensive VB Script and it allows you to convert the script in executable (exe). So what you can do is, write down the script to handle dialog box using AutoIt, convert it into executable and then call the executable when required. So let's see guys how to do it. But before we begin you need to download and install the Autoit. Download it from the following link
Download AutoIt
Handing Modal Dialogs
Modal Dialog box mean once it has appeared all the focus of that application will be on that dialog box only. You will not able to access any of the other component of parent window until you close the dialog box.
Some of the modal dialog boxes that we face frequently during web application testing are
Save Dialog Box
Save dialog box is bit different in IE and in Firefox. So I have write down two separate script for that.
AutoIt script to handle Firefox Save Dialog box
In Firefox when you click on the button/link to download a file. First it will ask you for you permission once you select yes, If you have selected to ask you for the path then only a dialog box will display.
If you will click on download link following dialogbox will appear in Firfox.
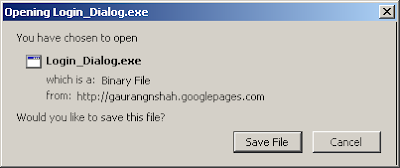
If you click "Save File" on above dialogbox this dialog box will apppears, Yeah if you have choose to ask where to save file from Option.
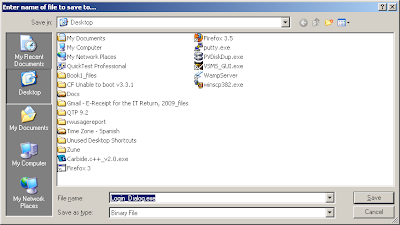
The executable and the script below will handle both of these dialogboxes. You just need to call it in proper way. Your need to pass arguments while calling the script or executable as following.
Save_Dialog_FF Operation FilePath
Operaion: Operation you want to perform when dialog box appears. Can be any of the following
cancel: Press Cancel on the dialog box
Save: Press the Save as Dialog box
FilePath: It's an option argument. It's a full path where you want to save the file.
i.e. C:\myfile.txt
AutoIt script to handle Internet Explorer Save Dialog box
Unlike Firefox dialog box internet explorers dialog box is bit different. First dialog box prompts with three choices Run, Save and cancel. The second dialog box is same as Firefox. If you click on the download link in the internet explorer following dialog box will display.
If you will click on "Save" button on the above dialog box Following dialog box will appear which will ask for the path to save the file.
The executable and the script below will handle both of these dialog boxes. You just need to call it in proper way. Your need to pass arguments while calling the script or executable as following. Save_Dialog_IE Operation FilePath Operaion: Operation you want to perform when dialog box appears. Can be any of the following Run: Clicks on the Run button. cancel: Press Cancel on the dialog box Save: Press the Save as Dialog box FilePath: It's an option argument. It's a full path where you want to save the file. i.e. C:\myfile.txt
Now you just need to copy and paste above scripts in Autoit IDE, convert those into executables, Save those to the project folder. And then just make a call to the executables with proper arguments, just before you execute the step which invokes the dialog box as shows here.
User Name and Password Dialog Box.
following is the AutoIt script to handle to User name and password dialog box.
That's because dialogs are native to the operating system you are running and selenium are designed to identify only web component.
After searching too much on the Internet and other forums, I finally got the solution to all my problems. The solution is too simple. As you can't handle the dialog box through selenium, use the other software to do this. And AutoIT is best at this.
Download Execuable (exe) to Handle Save Dialog of Firefox
Download Execuable (exe) to Handle Save Dialog of Internet Explorer
Download Execuable (exe) to Handle Authentication Dialog
AutoIt
Autoit is a free scripting language designed to automate the windows component. It's just like an extensive VB Script and it allows you to convert the script in executable (exe). So what you can do is, write down the script to handle dialog box using AutoIt, convert it into executable and then call the executable when required. So let's see guys how to do it. But before we begin you need to download and install the Autoit. Download it from the following link
Download AutoIt
Handing Modal Dialogs
Modal Dialog box mean once it has appeared all the focus of that application will be on that dialog box only. You will not able to access any of the other component of parent window until you close the dialog box.
Some of the modal dialog boxes that we face frequently during web application testing are
Save Dialog Box
Save dialog box is bit different in IE and in Firefox. So I have write down two separate script for that.
AutoIt script to handle Firefox Save Dialog box
In Firefox when you click on the button/link to download a file. First it will ask you for you permission once you select yes, If you have selected to ask you for the path then only a dialog box will display.
If you will click on download link following dialogbox will appear in Firfox.
If you click "Save File" on above dialogbox this dialog box will apppears, Yeah if you have choose to ask where to save file from Option.
The executable and the script below will handle both of these dialogboxes. You just need to call it in proper way. Your need to pass arguments while calling the script or executable as following.
Save_Dialog_FF Operation FilePath
Operaion: Operation you want to perform when dialog box appears. Can be any of the following
cancel: Press Cancel on the dialog box
Save: Press the Save as Dialog box
FilePath: It's an option argument. It's a full path where you want to save the file.
i.e. C:\myfile.txt
;--------------------------------------------------------- ;~ Save_Dialog_FF.au3 ;~ Purpose: To handle the Dowload/save Dialogbox in Firefox ;~ Usage: Save_Dialog_FF.exe "Dialog Title" "Opetaion" "Path" ;~ Create By: Gaurang Shah ;~ Email: gaurangnshah@gmail.com ;---------------------------------------------------------- ; set the select mode to select using substring AutoItSetOption("WinTitleMatchMode","2") if $CmdLine[0] < 2 then ; Arguments are not enough msgbox(0,"Error","Supply all the Arguments, Dialog title,Save/Cancel and Path to save(optional)") Exit EndIf ; wait until dialog box appears WinWait($CmdLine[1]) ; match the window with substring $title = WinGetTitle($CmdLine[1]) ; retrives whole window title WinActive($title); ; if user choose to save file If (StringCompare($CmdLine[2],"Save",0) = 0) Then WinActivate($title) WinWaitActive($title) Sleep(1) ; If firefox is set the save the file on some specific location without asking user. ;Save the File send("{TAB}") Send("{ENTER}") if ( StringCompare(WinGetTitle("[active]"),$title,0) = 0 ) Then WinActivate($title) send("{TAB}") Send("{ENTER}") EndIf ;if firefox is set to prompt user for save path. if WinExists("Enter name") Then $title = WinGetTitle("Enter name") if($CmdLine[0] = 2) Then ; If user hasn't provided path to save ;save to default path. WinActivate($title) ControlClick($title,"","Button2") Else ; If user has provided path ;Set path and save file WinActivate($title) WinWaitActive($title) ControlSetText($title,"","Edit1",$CmdLine[3]) ControlClick($title,"","Button2") EndIf Else ;Firefox is configured to save file at specific location Exit EndIf EndIf ; do not save the file If (StringCompare($CmdLine[2],"Cancel",0) = 0) Then WinWaitActive($title) Send("{ESCAPE}") EndIfDownload above script
AutoIt script to handle Internet Explorer Save Dialog box
Unlike Firefox dialog box internet explorers dialog box is bit different. First dialog box prompts with three choices Run, Save and cancel. The second dialog box is same as Firefox. If you click on the download link in the internet explorer following dialog box will display.
;-------------------------------------------------------------------- ;~ Save_Dialog_IE.au3 ;~ Purpose: To handle the Dowload/save Dialogbox in Internet Explorer ;~ Usage: Save_Dialog_IE.exe "Dialog Title" "Opetaion" "Path" ;~ Create By: Gaurang Shah ;~ Email: gaurangnshah@gmail.com ;-------------------------------------------------------------------- AutoItSetOption("WinTitleMatchMode","2") ; set the select mode to select using substring if $CmdLine[0] < 2 then ; Arguments are not enough msgbox(0,"Error","Supply all the arguments, Dialog title,Run/Save/Cancel and Path to save(optional)") Exit EndIf ; wait Until dialog box appears WinWait($CmdLine[1]) ; match the window with substring $title = WinGetTitle($CmdLine[1]) ; retrives whole window title WinActivate($title) If (StringCompare($CmdLine[2],"Run",0) = 0) Then WinActivate($title) ControlClick($title,"","Button1") EndIf If (StringCompare($CmdLine[2],"Save",0) = 0) Then WinWaitActive($title) ControlClick($title,"","Button2") ; Wait for the new dialogbox to open Sleep(2) WinWait("Save") $title = WinGetTitle("Save") ;$title = WinGetTitle("[active]") if($CmdLine[0] = 2) Then ;click on the save button WinWaitActive($title) ControlClick($title,"","Button2") Else ;Set path and save file WinWaitActive($title) ControlSetText($title,"","Edit1",$CmdLine[3]) ControlClick($title,"","Button2") EndIf EndIf If (StringCompare($CmdLine[2],"Cancel",0) = 0) Then WinWaitActive($title) ControlClick($title,"","Button3") EndIfDownload above script
Now you just need to copy and paste above scripts in Autoit IDE, convert those into executables, Save those to the project folder. And then just make a call to the executables with proper arguments, just before you execute the step which invokes the dialog box as shows here.
/** * @author Gaurang Shah * Purpose: Shows how to invoke Executable to handle dialog box * Email: gaurangnshah@gmail.com */ import org.testng.annotations.*; import com.thoughtworks.selenium.DefaultSelenium; import com.thoughtworks.selenium.Selenium; public class Handle_Dialogs { private Selenium selenium; @BeforeClass public void startSelenium() { selenium = new DefaultSelenium("localhost", 4444, "*chrome", "http://www.autoitscript.com/"); selenium.start(); } @AfterClass(alwaysRun=true) public void stopSelenium() { this.selenium.stop(); } @Test public void handleSaveDialog() throws Exception { String[] dialog; selenium.open("/site/autoit/downloads/"); selenium.waitForPageToLoad("3000"); selenium.click("//img[@alt='Download AutoIt']"); Thread.sleep(2000); String browser = selenium.getEval("navigator.userAgent"); if(browser.contains("IE")){ System.out.print("Browser= IE "+browser); dialog = new String[]{ "Save_Dialog_IE.exe","Download","Save" }; Runtime.getRuntime().exec(dialog); } if(browser.contains("Firefox")){ System.out.print("Browser= Firefox "+browser); dialog = new String[] { "Save_Dialog_FF.exe","Opening","save" }; Runtime.getRuntime().exec(dialog); } } }Download above File
User Name and Password Dialog Box.
following is the AutoIt script to handle to User name and password dialog box.
;~ ------------------------------------------------------------ ;~ Save_Dialog_IE.au3 ;~ To handle the Authentication Dialogbox ;~ Create By: Gaurang Shah ;~ Usage: Login_dialog.au3 "Dialog Title" "User Name" "Password" ;~ ------------------------------------------------------------ AutoItSetOption("WinTitleMatchMode","2") if $CmdLine[0] < 3 then msgbox(0,"Error","Supply all the Arguments, Dialog title User Name, Password") Exit EndIf WinWait($CmdLine[1]) ; match the window with substring $title = WinGetTitle($CmdLine[1]) ; retrives whole window title ControlSend($title,"","Edit2",$CmdLine[2]);Sets User Name ControlSend($title,"","Edit3",$CmdLine[3]);Sets Password ControlClick($title,"","OK");Download above script